Display Class Reference
Represents a display that can be used to display images. More...
Inheritance diagram for Display:
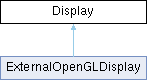
Public Types
using | WindowClosedHandler = std::function< void(Display &display)> Function prototype for window-closed event handlers. |
using | NotificationToken = void * Represents a registered callback. |
Public Member Functions
bool | displayBuffer (const std::shared_ptr< ic4::ImageBuffer > &buffer, Error &err=Error::Default()) Displays a specific image buffer. |
bool | setRenderPosition (DisplayRenderPosition pos, int left=-1, int top=-1, int width=-1, int height=-1, Error &err=Error::Default()) Configure the image scaling and alignment options for a display. |
DisplayStatistics | statistics (Error &err=Error::Default()) Queries display statistics. |
NotificationToken | eventAddWindowClosed (WindowClosedHandler cb, Error &err=Error::Default()) Registers a new window-closed event handler. |
bool | eventRemoveWindowClosed (NotificationToken token, Error &err=Error::Default()) Unregisters a window-closed event handler. |
Static Public Member Functions
static std::shared_ptr< Display > | create (DisplayType type, WindowHandle hParent, Error &err=Error::Default()) Creates a new display. |
Detailed Description
Represents a display that can be used to display images.
To create a display, call Display::create() or ExternalOpenGLDisplay::create().
Display objects are generally used in two distinct ways:
- The display is connected to a data stream when calling Grabber::streamSetup(), automatically displaying all images from the opened device.
- ImageBuffer objects are displayed manually by calling Display::displayBuffer().
Display objects are neither copyable nor movable, and are only handled via std::shared_ptr<Display>
.
- Note
- Some functions, such as Grabber::streamSetup(), takes shared ownership of the display object. The display is kept alive by the Grabber instance even if no external reference to the display object exists.
- See also
- Display::create
Member Typedef Documentation
◆ NotificationToken
using NotificationToken = void* |
Represents a registered callback.
When a callback function is registered using Display::eventAddWindowClosed, a token is returned.
The token can then be used to remove the callback using Display::eventRemoveWindowClosed at a later time.
◆ WindowClosedHandler
using WindowClosedHandler = std::function<void(Display& display)> |
Function prototype for window-closed event handlers.
- Parameters
-
[in] display The Display on which the event handler was registered.
Member Function Documentation
◆ create()
|
inlinestatic |
Creates a new display.
- Parameters
-
[in] type The type of display to create [in] hParent Handle to the parent window to embed the display into. [out] err Reference to an error handler. See Error Handling for details.
- Returns
- The new display, or
nullptr
if an error occurs.
- Warning
- This function only works in Windows platforms. For other platforms, use ExternalOpenGLDisplay::create().
◆ displayBuffer()
|
inline |
Displays a specific image buffer.
- Parameters
-
[in] buffer The buffer to display [out] err Reference to an error handler. See Error Handling for details.
- Returns
true
on success, otherwisefalse
.
- Remarks
- When buffer is
nullptr
, the display is cleared and will no longer display the previous buffer.
◆ eventAddWindowClosed()
|
inline |
Registers a new window-closed event handler.
- Parameters
-
[in] cb Callback function to be called when the display window is closed. [out] err Reference to an error handler. See Error Handling for details.
- Returns
- A token that can be used to unregister the callback using .\n If an error occurrs, the function returns
nullptr
.
- See also
- Display::eventRemoveWindowClosed
◆ eventRemoveWindowClosed()
|
inline |
Unregisters a window-closed event handler.
- Parameters
-
[in] token A token that was returned when registering an event handler using Display::eventAddWindowClosed(). [out] err Reference to an error handler. See Error Handling for details.
- Returns
true
on success, otherwisefalse
.
- See also
- Display::eventAddWindowClosed
◆ setRenderPosition()
|
inline |
Configure the image scaling and alignment options for a display.
- Parameters
-
[in] pos The scaling and alignment mode to use [in] left The left coordinate of the target rectangle inside the display window [in] top The top coordinate of the target rectangle inside the display window [in] width The width of the target rectangle inside the display window [in] height The height of the target rectangle inside the display window [out] err Reference to an error handler. See Error Handling for details.
- Returns
true
on success, otherwisefalse
.
- Remarks
- The left, top, width and height parameters are ignored unless mode is DisplayRenderPosition::Custom.
◆ statistics()
|
inline |
Queries display statistics.
- Parameters
-
[out] err Reference to an error handler. See Error Handling for details.
- Returns
- A DisplayStatistics structure containing the number of displayed and dropped frames.