PropEnumeration Class Reference
Enumeration properties represent a feature whose value is selected from a list of named entries. More...
Inheritance diagram for PropEnumeration:
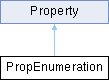
Public Member Functions
std::vector< PropEnumEntry > | entries (Error &err=Error::Default()) const Returns the list of entries in this enumeration property. |
PropEnumEntry | selectedEntry (Error &err=Error::Default()) const Returns the currently selected entry of this enumeration property. |
std::string | getValue (Error &err=Error::Default()) const Returns the name of the currently selected entry of this enumeration property. |
PropEnumEntry | findEntry (const std::string &name, Error &err=Error::Default()) const Finds the enumeration entry with a specified name. |
PropEnumEntry | findEntry (int64_t value, Error &err=Error::Default()) const Finds the enumeration entry with a specified value. |
bool | setIntValue (int64_t entry_value, Error &err=Error::Default()) Selects an enumeration entry by its value. |
int64_t | getIntValue (Error &err=Error::Default()) const Reads the value of the currently selected entry of this enumeration property. |
bool | setValue (const std::string &entry_name, Error &err=Error::Default()) Selects an enumeration entry by its name. |
bool | selectEntry (const PropEnumEntry &entry, Error &err=Error::Default()) Selects a specified enumeration entry. |
![]() | |
Property ()=default Creates an invalid object. |
|
bool | is_valid () const noexcept Checks whether this property is a valid object. |
bool | operator== (const Property &other) const noexcept Checks whether a property object refers to the same property as another property object. |
bool | operator!= (const Property &other) const noexcept Checks whether a property object refers to a different property as another property object. |
bool | operator< (const Property &other) const noexcept Provides an ordering of property objects. |
PropType | type (Error &err=Error::Default()) const Returns the type of the property. |
std::string | name (Error &err=Error::Default()) const Returns the name of the property. |
bool | isAvailable (Error &err=Error::Default()) const Checks whether a property is currently available. |
bool | isLocked (Error &err=Error::Default()) const Checks whether a property is currently locked. |
bool | isLikelyLockedByStream (Error &err=Error::Default()) const Tries to determine whether a property is locked because a data stream is active. |
bool | isReadOnly (Error &err=Error::Default()) const Checks whether a property is read-only. |
PropVisibility | visibility (Error &err=Error::Default()) const Returns a visibility hint for the property. |
std::string | displayName (Error &err=Error::Default()) const Returns the display name of the property. |
std::string | tooltip (Error &err=Error::Default()) const Returns a tooltip for the property. |
std::string | description (Error &err=Error::Default()) const Returns a description for the property. |
PropCommand | asCommand (Error &err=Error::Default()) const Converts this property into a PropCommand. |
PropInteger | asInteger (Error &err=Error::Default()) const Converts this property into a PropInteger. |
PropBoolean | asBoolean (Error &err=Error::Default()) const Converts this property into a PropBoolean. |
PropFloat | asFloat (Error &err=Error::Default()) const Converts this property into a PropFloat. |
PropString | asString (Error &err=Error::Default()) const Converts this property into a PropString. |
PropEnumeration | asEnumeration (Error &err=Error::Default()) const Converts this property into a PropEnumeration. |
PropEnumEntry | asEnumEntry (Error &err=Error::Default()) const Converts this property into a PropEnumEntry. |
PropRegister | asRegister (Error &err=Error::Default()) const Converts this property into a PropRegister. |
PropCategory | asCategory (Error &=Error::Default()) const Converts this property into a PropCategory. |
NotificationToken | eventAddNotification (NotificationHandler cb, Error &err=Error::Default()) Registers a new property notification event handler. |
bool | eventRemoveNotification (NotificationToken token, Error &err=Error::Default()) Unregisters a previously registered property notification event handler. |
bool | isSelector (Error &err=Error::Default()) Indicates whether this property's value changes the meaning and/or value of other properties. |
std::vector< Property > | selectedProperties (Error &err=Error::Default()) Returns the list of properties whose values' meaning depend on this property. |
Additional Inherited Members
![]() |
|
using | NotificationHandler = std::function< void(Property &prop)> Function prototype for property notification event handlers. |
using | NotificationToken = void * Represents a registered callback. |
Detailed Description
Enumeration properties represent a feature whose value is selected from a list of named entries.
Common examples for an enumeration properties are PixelFormat
, TriggerMode
or ExposureAuto
.
The value of an enumeration property can be get or set by both a enumeration entry's name or value.
Enumeration entries are represented by PropEnumEntry objects; a call to PropEnumeration::entries() returns the list of possible entries for an enumeration.
PropEnumeration instances are created in two ways:
- By converting a Property known to be a command property using Property::asEnumeration()
- By directly querying a typed known property using PropertyMap::findEnumeration().
Member Function Documentation
◆ entries()
|
inline |
Returns the list of entries in this enumeration property.
- Parameters
-
[out] err Reference to an error handler. See Error Handling for details.
- Returns
- A
std::vector
containing the enumeration entries.
If an error occurred, the function returns an empty vector. Check the err output parameter for details.
◆ findEntry() [1/2]
|
inline |
Finds the enumeration entry with a specified name.
- Parameters
-
[in] name The name of the requested enumeration entry [out] err Reference to an error handler. See Error Handling for details.
- Returns
- The enumeration entry whose name is equal to name.
If no matching entry is found, an invalid property object is returned.
Check Property::is_valid() or the err output parameter.
◆ findEntry() [2/2]
|
inline |
Finds the enumeration entry with a specified value.
- Parameters
-
[in] value The value of the requested enumeration entry [out] err Reference to an error handler. See Error Handling for details.
- Returns
- The enumeration entry whose value is equal to value.
If no matching entry is found, an invalid property object is returned.
Check Property::is_valid() or the err output parameter.
◆ getIntValue()
|
inline |
Reads the value of the currently selected entry of this enumeration property.
- Parameters
-
[out] err Reference to an error handler. See Error Handling for details.
- Returns
- The value of the currently selected entry, or
0
, if an error occurred.
Check the err output parameter for details.
- See also
- PropEnumeration::setIntValue()
◆ getValue()
|
inline |
Returns the name of the currently selected entry of this enumeration property.
- Parameters
-
[out] err Reference to an error handler. See Error Handling for details.
- Returns
- The name of the currently selected enumeration entry.
If an error occurred, an empty string is returned.
Check the the err output parameter for details.
◆ selectedEntry()
|
inline |
Returns the currently selected entry of this enumeration property.
- Parameters
-
[out] err Reference to an error handler. See Error Handling for details.
- Returns
- The currently selected enumeration entry.
If an error occurred, an invalid property object is returned.
Check Property::is_valid() or the err output parameter.
◆ selectEntry()
|
inline |
Selects a specified enumeration entry.
- Parameters
-
[in] entry The enumeration entry to to select [out] err Reference to an error handler. See Error Handling for details.
- Returns
true
on success, otherwisefalse
.
Check the err output parameter for details.
- See also
- PropEnumeration::selectedEntry()
◆ setIntValue()
|
inline |
Selects an enumeration entry by its value.
- Parameters
-
[in] entry_value The value of the entry to select [out] err Reference to an error handler. See Error Handling for details.
- Returns
true
on success, otherwisefalse
.
Check the err output parameter for details.
- See also
- PropEnumeration::intValue()
◆ setValue()
|
inline |
Selects an enumeration entry by its name.
- Parameters
-
[in] entry_name The name of the entry to to select [out] err Reference to an error handler. See Error Handling for details.
- Returns
true
on success, otherwisefalse
.
Check the err output parameter for details.
- See also
- PropEnumeration::getValue()
- PropEnumeration::setSelectedEntry()
- PropEnumeration::setIntValue()